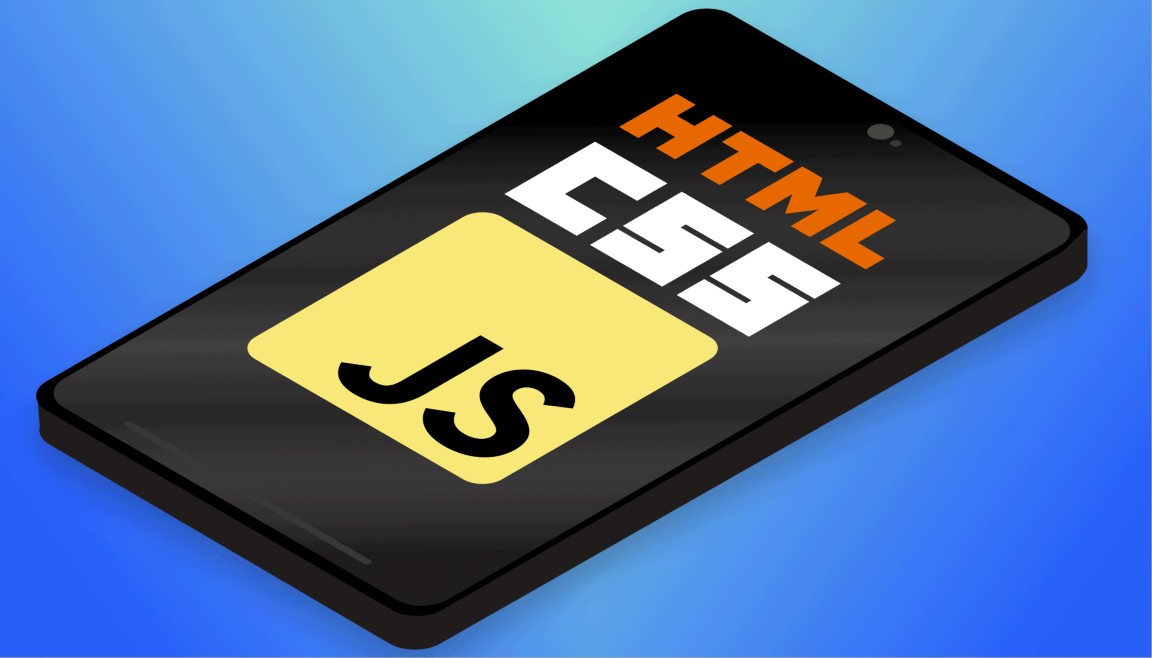
Master the core fundamentals of JavaScript, the language that powers modern websites and applications.
Whether you’re aiming for frontend development, WordPress customization, or full-stack programming, this beginner-friendly course will teach you how to think like a developer and build interactive, dynamic experiences from scratch.
Learn by doing with mini-projects, exercises, and simple real-world examples that bring each lesson to life.
JavaScript – The Language of the Web
The demand for skilled JavaScript developers continues to grow, making now the perfect time to launch or advance your career in web development. Whether you’re a complete beginner or looking to refine your skills, mastering JavaScript opens doors to exciting opportunities, from building dynamic front-end applications to developing full-stack solutions. With the right knowledge, you can create high-performance, scalable applications and become a sought-after web developer.
Recommended Courses:
Module 1: Introduction to JavaScript
- What JavaScript Is and Why It Matters
- How JavaScript Fits Into Websites (HTML + CSS + JS)
- Setting Up Your First JS Project (no frameworks!)
- Your First JavaScript Code (Hello, Console!)
🎯 Goal: Understand where JavaScript fits into the web ecosystem and run your first script.
Module 2: JavaScript Fundamentals
- Variables and Constants (
let
,const
,var
) - Data Types (Strings, Numbers, Booleans, Arrays, Objects)
- Basic Operators (math, comparison, logical)
🎯 Goal: Master the basic building blocks of all JS programs.
Module 3: Control Flow and Logic
- Conditional Statements (if/else, switch)
- Comparison and Logical Operators
- Truthy vs Falsy Values
🎯 Goal: Add decision-making ability to your code.
Module 4: Functions and Scope
- Writing and Calling Functions
- Function Parameters and Return Values
- Understanding Variable Scope (local vs global)
- Arrow Functions (
=>
) Basics
🎯 Goal: Write reusable blocks of code with confidence.
Module 5: Arrays and Loops
- Creating and Manipulating Arrays
- Common Array Methods (
push
,pop
,shift
,unshift
,slice
,splice
) - Looping Over Arrays (
for
,while
,forEach
)
🎯 Goal: Organize and process collections of data.
Module 6: Objects and the Basics of OOP
- What Are Objects in JavaScript?
- Object Properties and Methods
- Intro to the Concept of “this” in Objects
- Basic Constructor Functions (early OOP)
🎯 Goal: Understand how real-world items are modeled in code.
Module 7: The Document Object Model (DOM)
- What is the DOM and Why it Matters
- Selecting Elements (
querySelector
,getElementById
, etc.) - Manipulating HTML and CSS with JavaScript
- Adding and Removing Elements Dynamically
🎯 Goal: Control your web page with JavaScript interaction.
Module 8: Events and User Interaction
- What Events Are (click, submit, hover, keypress)
- Adding Event Listeners
- Event Objects and Event Handling
- Building Interactive UI Components (like modals, tabs)
🎯 Goal: Respond to user actions and make your websites interactive.
Module 9: Working with Forms and User Input
- Capturing Form Data
- Validating User Input with JavaScript
- Displaying Errors or Success Messages Dynamically
🎯 Goal: Master front-end form validation without plugins.
Module 10: Asynchronous JavaScript Basics
- Introduction to Asynchronous Programming
setTimeout
,setInterval
- Introduction to Fetch API (basic data fetching)
- Promises (conceptual intro)
🎯 Goal: Start understanding how modern websites handle real-time updates and external data.
Module 11: Building a Mini Project: Interactive To-Do List
- Create tasks, mark them complete, delete them
- Store tasks in browser local storage
- Make the UI dynamic and responsive
🎯 Goal: Apply everything learned to a working mini-app!
Module 12: JavaScript Best Practices
- Writing Clean, Readable Code
- Avoiding Common Beginner Mistakes
- Debugging with DevTools
- Structuring Code for Scalability
🎯 Goal: Code smarter, not harder — and learn professional habits.
Module 13: Next Steps and Beyond
- Where to Go After JS Fundamentals (DOM Projects, Frameworks like React)
- Prepare for Real-World Projects (APIs, Apps, CMS Integrations)
- Preparing for Advanced JavaScript and Full-Stack Paths
🎯 Goal: Set up a clear path to level up after the basics.
🏆 Outcomes After This Course
- Understand how JavaScript powers dynamic websites
- Build fully interactive frontend features from scratch
- Prepare for modern JavaScript libraries like React
- Have a working portfolio project (To-Do List app)
Project Details
Build a strong JavaScript foundation with essential concepts, best practices, and hands-on learning.